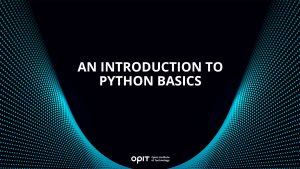
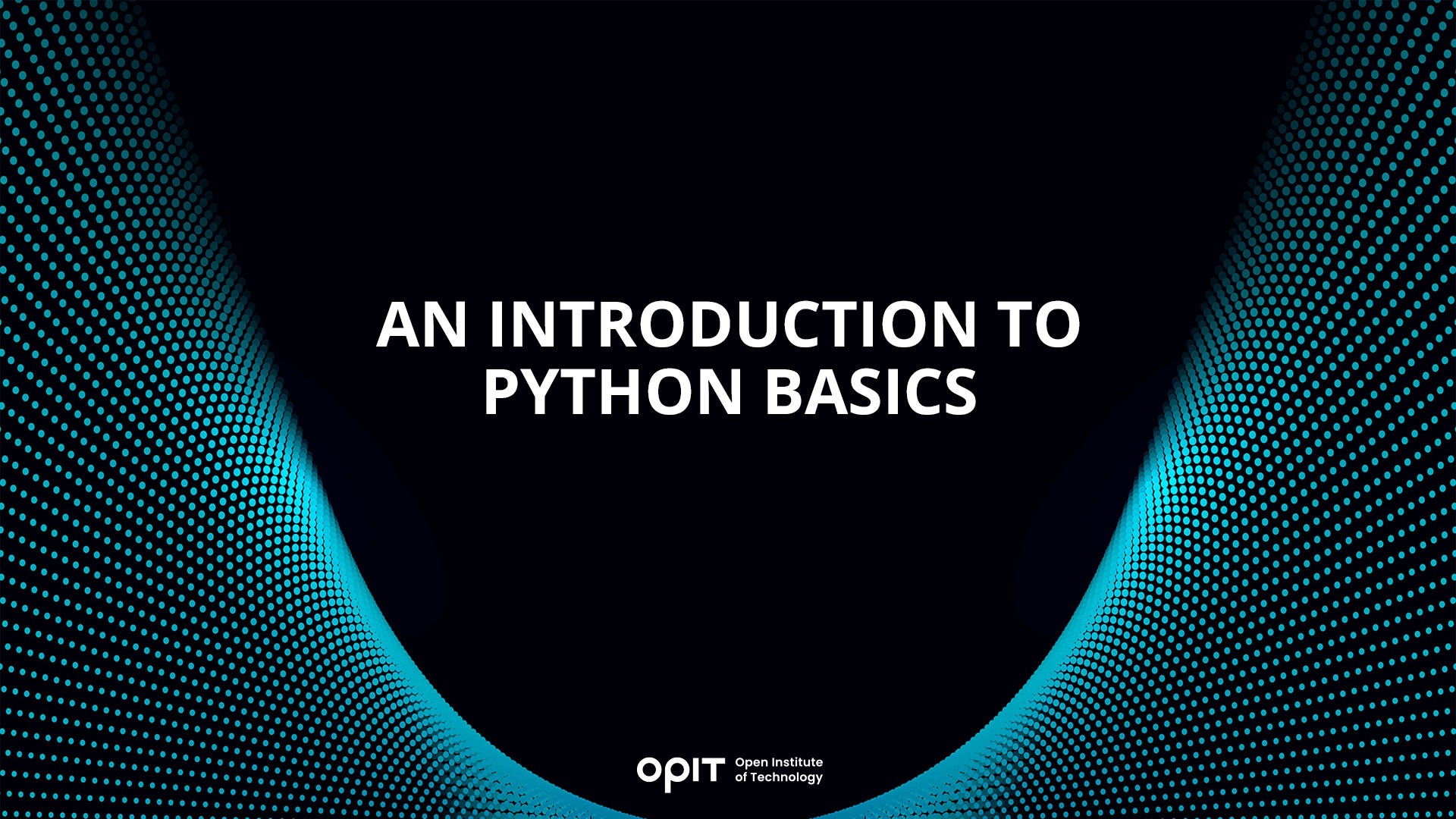
Just like the snake it’s named after, Python has wrapped itself around the programming world, becoming a deeply entrenched teaching and practical tool since its 1991 introduction. It’s one of the world’s most used programming languages, with Statista claiming that 48.07% of programmers use it, making it as essential as SQL, C, and even HTML to computer scientists.
This article serves as an introduction to Python programming for beginners. You’ll learn Python basics, such as how to install it and the concepts that underpin the language. Plus, we’ll show you some basic Python code you can use to have a little play around with the language.
Python Basics
It stands to reason that you need to download and install Python onto your system before you can start using it. The latest version of Python is always available at Python.org. Different versions are available for Windows, Linux, macOS, iOS, and several other machines and operating systems.
Installing Python is a universal process across operating systems. Download the installer for your OS from Python.org and open its executable. Follow the instructions and you should have Python up and running, and ready for you to play around with some Python language basics, in no time.
Python IDEs and Text Editors
Before you can start coding in your newly-installed version of Python, you need to install an integrated development environment (IDE) to your system. These applications are like a bridge between the language you write in and the visual representation of that language on your screen. But beyond being solely source code editors, many IDEs serve as debuggers, compilers, and even feature automation that can complete code (or at least offer suggestions) on your behalf.
Some of the best Python IDEs include:
- Atom
- Visual Studio
- Eclipse
- PyCharm
- Komodo IDE
But there are plenty more besides. Before choosing an IDE, ask yourself the following questions to determine if the IDE you’re considering is right for your Python project:
- How much does it cost?
- Is it easy to use?
- What are its debugging and compiling features?
- How fast is the IDE?
- Does this IDE give me access to the libraries I’ll need for my programs?
Basic Python Concepts
Getting to grips with the Python basics for beginners starts with learning the concepts that underpin the language. Each of these concepts defines actions you can take in the language, meaning they’re essentially for writing even the simplest of programs.
Variables and Data Types
Variables in Python work much like they do for other programming languages – they’re containers in which you store a data value. The difference between Python and other languages is that Python doesn’t have a specific command used to declare a variable. Instead, you create a variable the moment you assign a value to a data type.
As for data types, they’re split into several categories, with most having multiple sub-types you can use to define different variables:
- String – “str”
- Numeric – “int,” “complex,” “float”
- Sequence – “list,” “range,” “tuple”
- Boolean – “bool”
- Binary – “memoryview,” “bytes,” “bytearray”
There are more, though the above should be enough for your Python basics notes. Each of these data types serves a different function. For example, on the numerical side, “int” allows you to store signed integers of no defined length, while “float” lets you assign decimals up to 15 points.
Operators
When you have your variables and values, you’ll use operators to perform actions using them. These actions range from the simple (adding and subtracting numbers) to the complex (comparing values to each other). Though there are many types of operators you’ll learn as you venture beyond the Python language basics, the following three are some of the most important for basic programs:
- Arithmetic operators – These operators allow you to handle most aspects of basic math, including addition, subtraction, division, and multiplication. There are also arithmetic operators for more complex operations, including floor division and exponentiation.
- Comparison operators – If you want to know which value is bigger, comparison operators are what you use. They take two values, compare them, and give you a result based on the operator’s function.
- Logical operators – “And,” “Or,” and “Not” are your logical operators and they combine to form conditional statements that give “True” or “False”
Control Structures
As soon as you start introducing different types of inputs into your code, you need control structures to keep everything organized. Think of them as the foundations of your code, directing variables to where they need to go while keeping everything, as the name implies, under control. Two of the most important control structures are:
- Conditional Statements – “If,” “Else,” and “elif” fall into this category. These statements basically allow you to determine what the code does “if” something is the case (such as a variable equaling a certain number) and what “else” to do if the condition isn’t met.
- Loops – “For” and “while” are your loop commands, with the former being used to create an iterative sequence, with the latter setting the condition for that sequence to occur.
Functions
You likely don’t want every scrap of code you write to run as soon as you start your program. Some chunks (called functions) should only run when they’re called by other parts of the code. Think of it like giving commands to a dog. A function will only sit, stay, or roll over when another part of the code tells it to do what it does.
You need to define and call functions.
Use the “def” keyword to define a function, as you see in the following example:
def first_function():
print (“This is my first function”)
When you need to call that function, you simply type the function’s name followed by the appropriate parenthesis:
first_function()
That “call” tells your program to print out the words “This is my first function” on the screen whenever you use it.
Interestingly, Python has a collection of built-in functions, which are functions included in the language that anybody can call without having to first define the function. Many relate to the data types discussed earlier, with functions like “str()” and “int()” allowing you to define strings and integers respectively.
Python – Basic Programs
Now that you’ve gotten to grips with some of the Python basics for beginners, let’s look at a few simple programs that almost anybody can run.
Hello, World! Program
The starting point for any new coder in almost any new language is to get the screen to print out the words “Hello, World!”. This one is as simple as you can get, as you’ll use the print command to get a piece of text to appear on screen:
print(‘Hello, World! ‘)
Click what “Run” button in your IDE of choice and you’ll see the words in your print command pop up on your monitor. Though this is all simple enough, make sure you make note of the use of the apostrophes/speech mark around the text. If you don’t have them, your message doesn’t print.
Basic Calculator Program
Let’s step things up with one of the Python basic programs for beginners that helps you to get to grips with functions. You can create a basic calculator using the language by defining functions for each of your arithmetic operators and using conditional statements to tell the calculator what to do when presented with different options.
The following example comes from Programiz.com:
# This function adds two numbers
def add(x, y):
return x + y
# This function subtracts two numbers
def subtract(x, y):
return x – y
# This function multiplies two numbers
def multiply(x, y):
return x * y
# This function divides two numbers
def divide(x, y):
return x / y
print(“Select operation.”)
print(“1.Add”)
print(“2.Subtract”)
print(“3.Multiply”)
print(“4.Divide”)
while True:
# Take input from the user
choice = input(“Enter choice(1/2/3/4): “)
# Check if choice is one of the four options
if choice in (‘1’, ‘2’, ‘3’, ‘4’):
try:
num1 = float(input(“Enter first number: “))
num2 = float(input(“Enter second number: “))
except ValueError:
print(“Invalid input. Please enter a number.”)
continue
if choice == ‘1’:
print(num1, “+”, num2, “=”, add(num1, num2))
elif choice == ‘2’:
print(num1, “-“, num2, “=”, subtract(num1, num2))
elif choice == ‘3’:
print(num1, “*”, num2, “=”, multiply(num1, num2))
elif choice == ‘4’:
print(num1, “/”, num2, “=”, divide(num1, num2))
# Check if user wants another calculation
# Break the while loop if answer is no
next_calculation = input(“Let’s do next calculation? (yes/no): “)
if next_calculation == “no”:
break
else:
print(“Invalid Input”)
When you run this code, your executable asks you to choose a number between 1 and 4, with your choice denoting which mathematical operator you wish to use. Then, you enter your values for “x” and “y”, with the program running a calculation between those two values based on the operation choice. There’s even a clever piece at the end that asks you if you want to run another calculation or cancel out of the program.
Simple Number Guessing Game
Next up is a simple guessing game that takes advantage of the “random” module built into Python. You use this module to generate a number between 1 and 99, with the program asking you to guess which number it’s chosen. But unlike when you play this game with your sibling, the number doesn’t keep changing whenever you guess the right answer.
This code comes from Python for Beginners:
import random
n = random.randint(1, 99)
guess = int(input(“Enter an integer from 1 to 99: “))
while True:
if guess < n:
print (“guess is low”)
guess = int(input(“Enter an integer from 1 to 99: “))
elif guess > n:
print (“guess is high”)
guess = int(input(“Enter an integer from 1 to 99: “))
else:
print (“you guessed it right! Bye!”)
break
Upon running the code, your program uses the imported “random” module to pick its number and then asks you to enter an integer (i.e., a whole number) between 1 and 99. You keep guessing until you get it right and the program delivers a “Bye” message.
Python Libraries and Modules
As you move beyond the basic Python language introduction and start to develop more complex code, you’ll find your program getting a bit on the heavy side. That’s where modules come in. You can save chunks of your code into a module, which is a file with the “.py” extension, allowing you to call that module into another piece of code.
Typically, these modules contain functions, variables, and classes that you want to use at multiple points in your main program. Retyping those things at every instance where they’re called takes too much time and leaves you with code that’s bogged down in repeated processes.
Libraries take things a step further by offering you a collection of modules that you can call from as needed, similar to how you can borrow any book from a physical library. Examples include the “Mayplotlib” library, which features a bunch of modules for data visualization, and “Beautiful Soup,” which allows you to extract data from XML and HTML files.
Best Practices and Tips for Basic Python Programs for Beginners
Though we’ve focused primarily on the code aspect of the language in these Python basic notes so far, there are a few tips that will help you create better programs that aren’t directly related to learning the language:
- Write clean code – Imagine that you’re trying to find something you need in a messy and cluttered room. It’s a nightmare to find what you’re looking for because you’re constantly tripping over stuff you don’t need. That’s what happens in a Python program if you create bloated code or repeat functions constantly. Keep it clean and your code is easier to use.
- Debugging and error handling – Buggy code is frustrating to users, especially if that code just dumps them out of a program when it hits an error. Beyond debugging (which everybody should do as standard) you must build error responses into your Python code to let users know what’s happening when something goes wrong.
- Use online communities and resources – Python is one of the most established programming languages in the world, and there’s a massive community built up around it. Take advantage of those resources. Try your hand at a program first, then take it to the community to see if they can point you in the right direction.
Get to Grips With the Basic Concepts of Python
With these Python introduction notes, you have everything you need to understand some of the more basic aspects of the language, as well as run a few programs. Experimentation is your friend, so try taking what you’ve learned here and writing a few other simple programs for yourself. Remember – the Python community (along with stacks of online resources) are available to help you when you’re struggling.
Related posts
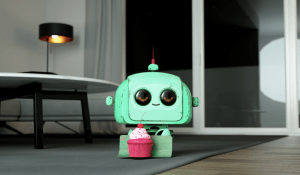
Source:
- The Yuan, Published on October 25th, 2024.
By Zorina Alliata
ALEXANDRIA, VIRGINIA – In recent years, artificial intelligence (AI) has grown and developed into something much bigger than most people could have ever expected. Jokes about robots living among humans no longer seem so harmless, and the average person began to develop a new awareness of AI and all its uses. Unfortunately, however – as is often a human tendency – people became hyper-fixated on the negative aspects of AI, often forgetting about all the good it can do. One should therefore take a step back and remember that humanity is still only in the very early stages of developing real intelligence outside of the human brain, and so at this point AI is almost like a small child that humans are raising.
AI is still developing, growing, and adapting, and like any new tech it has its drawbacks. At one point, people had fears and doubts about electricity, calculators, and mobile phones – but now these have become ubiquitous aspects of everyday life, and it is not difficult to imagine a future in which this is the case for AI as well.
The development of AI certainly comes with relevant and real concerns that must be addressed – such as its controversial role in education, the potential job losses it might lead to, and its bias and inaccuracies. For every fear, however, there is also a ray of hope, and that is largely thanks to people and their ingenuity.
Looking at education, many educators around the world are worried about recent developments in AI. The frequently discussed ChatGPT – which is now on its fourth version – is a major red flag for many, causing concerns around plagiarism and creating fears that it will lead to the end of writing as people know it. This is one of the main factors that has increased the pessimistic reporting about AI that one so often sees in the media.
However, when one actually considers ChatGPT in its current state, it is safe to say that these fears are probably overblown. Can ChatGPT really replace the human mind, which is capable of so much that AI cannot replicate? As for educators, instead of assuming that all their students will want to cheat, they should instead consider the options for taking advantage of new tech to enhance the learning experience. Most people now know the tell-tale signs for identifying something that ChatGPT has written. Excessive use of numbered lists, repetitive language and poor comparison skills are just three ways to tell if a piece of writing is legitimate or if a bot is behind it. This author personally encourages the use of AI in the classes I teach. This is because it is better for students to understand what AI can do and how to use it as a tool in their learning instead of avoiding and fearing it, or being discouraged from using it no matter the circumstances.
Educators should therefore reframe the idea of ChatGPT in their minds, have open discussions with students about its uses, and help them understand that it is actually just another tool to help them learn more efficiently – and not a replacement for their own thoughts and words. Such frank discussions help students develop their critical thinking skills and start understanding their own influence on ChatGPT and other AI-powered tools.
By developing one’s understanding of AI’s actual capabilities, one can begin to understand its uses in everyday life. Some would have people believe that this means countless jobs will inevitably become obsolete, but that is not entirely true. Even if AI does replace some jobs, it will still need industry experts to guide it, meaning that entirely new jobs are being created at the same time as some older jobs are disappearing.
Adapting to AI is a new challenge for most industries, and it is certainly daunting at times. The reality, however, is that AI is not here to steal people’s jobs. If anything, it will change the nature of some jobs and may even improve them by making human workers more efficient and productive. If AI is to be a truly useful tool, it will still need humans. One should remember that humans working alongside AI and using it as a tool is key, because in most cases AI cannot do the job of a person by itself.
Is AI biased?
Why should one view AI as a tool and not a replacement? The main reason is because AI itself is still learning, and AI-powered tools such as ChatGPT do not understand bias. As a result, whenever ChatGPT is asked a question it will pull information from anywhere, and so it can easily repeat old biases. AI is learning from previous data, much of which is biased or out of date. Data about home ownership and mortgages, e.g., are often biased because non-white people in the United States could not get a mortgage until after the 1960s. The effect on data due to this lending discrimination is only now being fully understood.
AI is certainly biased at times, but that stems from human bias. Again, this just reinforces the need for humans to be in control of AI. AI is like a young child in that it is still absorbing what is happening around it. People must therefore not fear it, but instead guide it in the right direction.
For AI to be used as a tool, it must be treated as such. If one wanted to build a house, one would not expect one’s tools to be able to do the job alone – and AI must be viewed through a similar lens. By acknowledging this aspect of AI and taking control of humans’ role in its development, the world would be better placed to reap the benefits and quash the fears associated with AI. One should therefore not assume that all the doom and gloom one reads about AI is exactly as it seems. Instead, people should try experimenting with it and learning from it, and maybe soon they will realize that it was the best thing that could have happened to humanity.
Read the full article below:
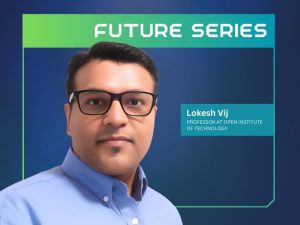
Source:
- The European Business Review, Published on October 27th, 2024.
By Lokesh Vij
Lokesh Vij is a Professor of BSc in Modern Computer Science & MSc in Applied Data Science & AI at Open Institute of Technology. With over 20 years of experience in cloud computing infrastructure, cybersecurity and cloud development, Professor Vij is an expert in all things related to data and modern computer science.
In today’s rapidly evolving technological landscape, the fields of blockchain and cloud computing are transforming industries, from finance to healthcare, and creating new opportunities for innovation. Integrating these technologies into education is not merely a trend but a necessity to equip students with the skills they need to thrive in the future workforce. Though both technologies are independently powerful, their potential for innovation and disruption is amplified when combined. This article explores the pressing questions surrounding the inclusion of blockchain and cloud computing in education, providing a comprehensive overview of their significance, benefits, and challenges.
The Technological Edge and Future Outlook
Cloud computing has revolutionized how businesses and individuals’ access and manage data and applications. Benefits like scalability, cost efficiency (including eliminating capital expenditure – CapEx), rapid innovation, and experimentation enable businesses to develop and deploy new applications and services quickly without the constraints of traditional on-premises infrastructure – thanks to managed services where cloud providers manage the operating system, runtime, and middleware, allowing businesses to focus on development and innovation. According to Statista, the cloud computing market is projected to reach a significant size of Euro 250 billion or even higher by 2028 (from Euro 110 billion in 2024), with a substantial Compound Annual Growth Rate (CAGR) of 22.78%. The widespread adoption of cloud computing by businesses of all sizes, coupled with the increasing demand for cloud-based services and applications, fuels the need for cloud computing professionals.
Blockchain, a distributed ledger technology, has paved the way by providing a secure, transparent, and tamper-proof way to record transactions (highly resistant to hacking and fraud). In 2021, European blockchain startups raised $1.5 billion in funding, indicating strong interest and growth potential. Reports suggest the European blockchain market could reach $39 billion by 2026, with a significant CAGR of over 47%. This growth is fueled by increasing adoption in sectors like finance, supply chain, and healthcare.
Addressing the Skills Gap
Reports from the World Economic Forum indicate that 85 million jobs may be displaced by a shift in the division of labor between humans and machines by 2025. However, 97 million new roles may emerge that are more adapted to the new division of labor between humans, machines, and algorithms, many of which will require proficiency in cloud computing and blockchain.
Furthermore, the World Economic Forum predicts that by 2027, 10% of the global GDP will be tokenized and stored on the blockchain. This massive shift means a surge in demand for blockchain professionals across various industries. Consider the implications of 10% of the global GDP being on the blockchain: it translates to a massive need for people who can build, secure, and manage these systems. We’re talking about potentially millions of jobs worldwide.
The European Blockchain Services Infrastructure (EBSI), an EU initiative, aims to deploy cross-border blockchain services across Europe, focusing on areas like digital identity, trusted data sharing, and diploma management. The EU’s MiCA (Crypto-Asset Regulation) regulation, expected to be fully implemented by 2025, will provide a clear legal framework for crypto-assets, fostering innovation and investment in the blockchain space. The projected growth and supportive regulatory environment point to a rising demand for blockchain professionals in Europe. Developing skills related to EBSI and its applications could be highly advantageous, given its potential impact on public sector blockchain adoption. Understanding the MiCA regulation will be crucial for blockchain roles related to crypto-assets and decentralized finance (DeFi).
Furthermore, European businesses are rapidly adopting digital technologies, with cloud computing as a core component of this transformation. GDPR (Data Protection Regulations) and other data protection laws push businesses to adopt secure and compliant cloud solutions. Many European countries invest heavily in cloud infrastructure and promote cloud adoption across various sectors. Artificial intelligence and machine learning will be deeply integrated into cloud platforms, enabling smarter automation, advanced analytics, and more efficient operations. This allows developers to focus on building applications without managing servers, leading to faster development cycles and increased scalability. Processing data closer to the source (like on devices or local servers) will become crucial for applications requiring real-time responses, such as IoT and autonomous vehicles.
The projected growth indicates a strong and continuous demand for blockchain and cloud professionals in Europe and worldwide. As we stand at the “crossroads of infinity,” there is a significant skill shortage, which will likely increase with the rapid adoption of these technologies. A 2023 study by SoftwareOne found that 95% of businesses globally face a cloud skills gap. Specific skills in high demand include cloud security, cloud-native development, and expertise in leading cloud platforms like AWS, Azure, and Google Cloud. The European Commission’s Digital Economy and Society Index (DESI) highlights a need for improved digital skills in areas like blockchain to support the EU’s digital transformation goals. A 2023 report by CasperLabs found that 90% of businesses in the US, UK, and China adopt blockchain, but knowledge gaps and interoperability challenges persist.
The Role of Educational Institutions
This surge in demand necessitates a corresponding increase in qualified individuals who can design, implement, and manage cloud-based and blockchain solutions. Educational institutions have a critical role to play in bridging this widening skills gap and ensuring a pipeline of talent ready to meet the demands of this burgeoning industry.
To effectively prepare the next generation of cloud computing and blockchain experts, educational institutions need to adopt a multi-pronged approach. This includes enhancing curricula with specialized programs, integrating cloud and blockchain concepts into existing courses, and providing hands-on experience with leading technology platforms.
Furthermore, investing in faculty development to ensure they possess up-to-date knowledge and expertise is crucial. Collaboration with industry partners through internships, co-teach programs, joint research projects, and mentorship programs can provide students with invaluable real-world experience and insights.
Beyond formal education, fostering a culture of lifelong learning is essential. Offering continuing education courses, boot camps, and online resources enables professionals to upskill or reskill and stay abreast of the latest advancements in cloud computing. Actively promoting awareness of career paths and opportunities in this field and facilitating connections with potential employers can empower students to thrive in the dynamic and evolving landscape of cloud computing and blockchain technologies.
By taking these steps, educational institutions can effectively prepare the young generation to fill the skills gap and thrive in the rapidly evolving world of cloud computing and blockchain.
Read the full article below:
Have questions?
Visit our FAQ page or get in touch with us!
Write us at +39 335 576 0263
Get in touch at hello@opit.com
Talk to one of our Study Advisors
We are international
We can speak in: